PlatformService & Operations
The concept behind a PlatformService is the idea to expose some native functionality, each one called FlutterOperation to Flutter.
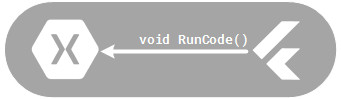
You only need to create a simple C# class or interface, declaring or implementing you desiderated methods and marking with appropriate attributes. If you need to manage some custom error, you can implement your custom PlatformOperationException.
Example
I want to provide a token to Flutter, in form of string value.
To do this i will create a TokenService class with the corrisponding GetToken() public method.
TokenService.cs
using Flutnet.ServiceModel;
namespace my.library
{
[PlatformService]
public class TokenService
{
[PlatformOperation]
public string GetToken()
{
return "YOUR_TOKEN";
}
}
}
In case you need to implement different logics for the Android and iOS, you can expose to flutter an interface and implement it on your specific projects.
ITokenService.cs
using Flutnet.ServiceModel;
namespace my.library
{
[PlatformService]
public interface ITokenService
{
[PlatformOperation]
public string GetToken();
}
}
Main Thread Required
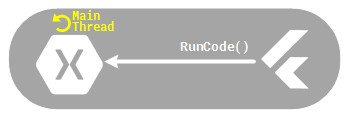
Based on the platform (Android or iOS), you may need to run some code on the Main Thread. Flutnet allow you to specify this requirement on the PlatformOperation attribute as properties.
MyService.cs
using Flutnet.ServiceModel;
namespace my.library
{
[PlatformService]
public class MyService
{
[PlatformOperation ( IosMainThreadRequired = true, AndroidMainThreadRequired = false ) ]
public void MyNativeCall();
}
}
In this example the operation MyNativeCall will be executed on the Main thread only for iOS. By default all the platform calls will be executed in background to improve UI performance.
Async support
Platform operations can be asyncronus, but only returning Task or Task<T>, where T is a supported type or PlatformData.
Note that void async is NOT supported. Use Task async instead.