Flutnet Bridge
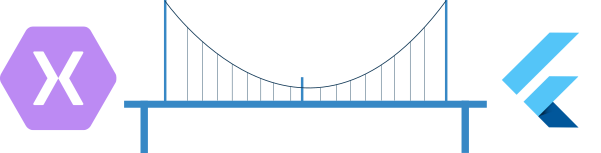
FlutnetBridge is one of most important core for Flutnet. This component allow Xamarin and Flutter to communicate in two different mode:
The first (PlatformChannel) is the standard communication mode for an Flutter module embedded in an existing application. The second (WebSocket) allows to run Xamarin and Flutter separate, allowing to modify the UI in flutter without rebuilding all the Xamarin application: should be very helpful in some cases, especially if you have already implemented all the Xamarin Service Operations and you want to concentrate on the UI development.
Note that WebSocket configuration is intended only for developing purpose. The Release build process will force the configuration to be PlatformChannel for both Xamarin and Flutter.
In debug the configuration should be the same for both Xamarin and Flutter project, otherwise the FlutnetBridge will not work properly.
The configuration is governed by Xamarin, and Flutter need to be aligned to it.
Flutter Embedding
Before diving in the FlutnetBridge configuration, we need to understand the meaning of Flutter Embedding.
A Flutter "module" is a project prepared to be embedded inside an existing Android or iOS app, so the host application (Xamarin in our case) need to reference a compiled version of it in order to be capable executing the module inside its environment.
The Flutter "module" project can even run "as a standalone application", so you can develop this part independently from the final host application. When you have finish, you just need to build the module before incorporating it inside the target host app.
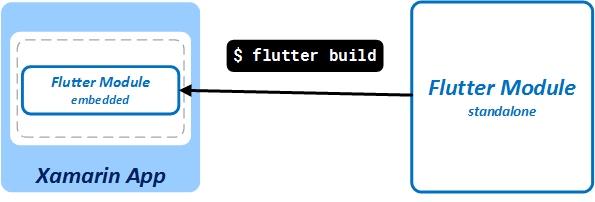
This premise was necessary to understand the next statement:
The embedded Flutter module can work only using the PlatformChannel configuration. Every changes in the flutter module require a compilation in order to update it in Xamarin project.
This is the main reason why Flutnet have introduced the WebSocket configuration in order to reduce the time development.
Note that if you want to run the Xamarin App for the first time, you need to build at list one time the Flutter module.
PlatformChannel mode
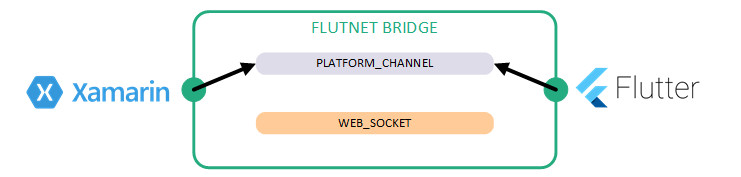
This mode is the standard way to provide communication between Flutter and your native application (Xamarin in our case). This configuration relies on the fact that the Flutter module is embedded inside your Xamarin application.
1 Application = Xamarin with Fluttermodule
Common Debug configuration error
In case the Flutter module was previously compiled using WebSocket configuration, all his platform call will be blocked by the FlutnetBridge.
To obtain this configuration, you need to configure both Xamarin and Flutter project.
Flutter module project
main.dart
import 'package:flutter/material.dart';
import 'package:my_first_app_bridge/flutnet_bridge.dart';
void main() {
// Configure the bridge mode for debug
FlutnetBridgeConfig.mode = FlutnetBridgeMode.PlatformChannel;
runApp(MyApp());
}
Xamarin Android
App.cs
using System;
using Android.App;
using Android.Content;
using Android.OS;
using Flutnet;
using Flutnet.Interop.Embedding.Engine;
using Environment = System.Environment;
using MyFirstApp.ServiceLibrary;
namespace MyFirstApp
{
// Singleton class for Application wide objects.
public static class App
{
// ...........
public static void ConfigureFlutnetBridge(FlutterEngine flutterEngine)
{
// Start the bridge: you can enable / disable websocket connection
try
{
#if DEBUG
_bridge = new FlutnetBridge(flutterEngine, AppContext, FlutnetBridgeMode.PlatformChannel);
#else
_bridge = new FlutnetBridge(flutterEngine, AppContext);
#endif
}
catch (Exception e)
{
// Handle bridge errors
}
}
// .............
}
}
Xamarin iOS
ViewController.cs
using System;
using UIKit;
using Flutnet;
using Flutnet.Interop;
using MyFirstApp.ServiceLibrary;
using System.Threading.Tasks;
namespace MyFirstApp
{
public partial class ViewController : FlutterViewController
{
// ........
public override async void ViewDidAppear(bool animated)
{
base.ViewDidAppear(animated);
// .......
try
{
// .......
// Initialize the bridge
#if (DEBUG)
_bridge = new FlutnetBridge(this.Engine, FlutnetBridgeMode.PlatformChannel);
#else
_bridge = new FlutnetBridge(this.Engine);
#endif
_initialized = true;
}
catch (Exception e)
{
// Handle Bridge errors
}
}
}
}
WebSocket mode
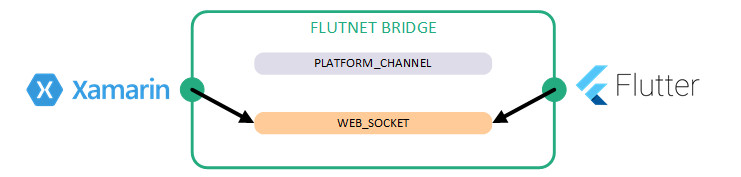
This mode is intended only for developing purposes in order to simplify the life of programmers (mostly). This configuration relies on the fact that the Flutter module run standalone outside your Xamarin application.
2 Applications = Xamarin + Flutter standalone
With the term "Flutter standalone" we just intend the Flutter module project started as a standlone application. This is done only for developing purpose. Remember that a Flutter module project is intended to be encapsulated inside an existing application.
To obtain this configuration, you need to configure both Xamarin and Flutter project.
Flutter module project
main.dart
import 'package:flutter/material.dart';
import 'package:my_first_app_bridge/flutnet_bridge.dart';
void main() {
// Configure the bridge mode for debug
FlutnetBridgeConfig.mode = FlutnetBridgeMode.WebSocket;
runApp(MyApp());
}
Xamarin Android
App.cs
using System;
using Android.App;
using Android.Content;
using Android.OS;
using Flutnet;
using Flutnet.Interop.Embedding.Engine;
using Environment = System.Environment;
using MyFirstApp.ServiceLibrary;
namespace MyFirstApp
{
// Singleton class for Application wide objects.
public static class App
{
// ...........
public static void ConfigureFlutnetBridge(FlutterEngine flutterEngine)
{
// Start the bridge: you can enable / disable websocket connection
try
{
#if DEBUG
_bridge = new FlutnetBridge(flutterEngine, AppContext, FlutnetBridgeMode.WebSocket);
#else
_bridge = new FlutnetBridge(flutterEngine, AppContext);
#endif
}
catch (Exception e)
{
// Handle bridge errors
}
}
// .............
}
}
Xamarin iOS
ViewController.cs
using System;
using UIKit;
using Flutnet;
using Flutnet.Interop;
using MyFirstApp.ServiceLibrary;
using System.Threading.Tasks;
namespace MyFirstApp
{
public partial class ViewController : FlutterViewController
{
// ........
public override async void ViewDidAppear(bool animated)
{
base.ViewDidAppear(animated);
// .......
try
{
// .......
// Start the bridge: you can enable / disable websocket connection
#if (DEBUG)
_bridge = new FlutnetBridge(this.Engine, FlutnetBridgeMode.WebSocket);
#else
_bridge = new FlutnetBridge(this.Engine);
#endif
_initialized = true;
}
catch (Exception e)
{
// Handle Bridge errors
}
}
}
}
This configuration will have two separated application running simultaneously, so the best practice is to run at first the Xamarin application (as back-end), and at second Flutter (as user interface).