Develop your user interface in Flutter
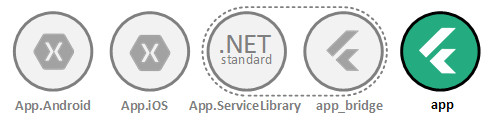
This section will not cover all the aspect about the flutter app, integrating the flutter video_player plugin to render a video from an URL.
The first thing to do is add the plugin needed by Flutter: open the pubspec.yaml and add this lines under "dependencies".
pubspec.yaml
....
dependencies:
flutter:
sdk: flutter
video_player: ^0.10.11+1
....
Follow the video_player readme section to configure the application permissions required by the plugin.
Because we are developing a flutter module, the execution host will be the Xamarin application, so we need to request the corrisponding plugins permissions in the Xamarin.Android and Xamarin.iOS applications, editing the corrisponding AndroidManifest.xml and Info.plist.
To run the Flutter module in STANDALONE, we need to edit the AndroidManifest.xml and Info.plist in the corrisponding temp folders .android and .ios.
Remember that every time you call $flutter clean command in the flutter module directory, this folders will be regenerated, so this changes should be redone.
Next, we will define the screen (with the video player) editing the main.dart file.
main.dart
import 'package:video_player/video_player.dart';
import 'package:flutter/material.dart';
void main() => runApp(VideoApp());
class VideoApp extends StatefulWidget {
@override
_VideoAppState createState() => _VideoAppState();
}
class _VideoAppState extends State<VideoApp> {
VideoPlayerController _controller;
@override
void initState() {
super.initState();
_controller = VideoPlayerController.network(
'https://flutter.github.io/assets-for-api-docs/assets/videos/bee.mp4')
..setLooping(true)
..initialize().then((_) {
// Ensure the first frame is shown after the video is initialized, even before the play button has been pressed.
setState(() {});
});
}
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Video Demo',
home: Scaffold(
body: Center(
child: _controller.value.initialized
? AspectRatio(
aspectRatio: _controller.value.aspectRatio,
child: VideoPlayer(_controller),
)
: Container(),
),
floatingActionButton: FloatingActionButton(
onPressed: () {
setState(() {
_controller.value.isPlaying
? _controller.pause()
: _controller.play();
});
},
child: Icon(
_controller.value.isPlaying ? Icons.pause : Icons.play_arrow,
),
),
),
);
}
@override
void dispose() {
super.dispose();
_controller.dispose();
}
}
Screenshots