Flutnet Runtime
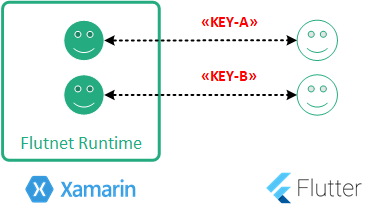
The Flutnet Runtime is the place where you need to register your PlatformService in order to access it from Flutter. The runtime need to be initialized with a key (commonly the name of the app), and each Service instance need to be registered with an unique key (string). This key is used to initialize the same instance class from Flutter.
Xamarin Android
App.cs
using System;
using Android.App;
using Android.Content;
using Android.OS;
using Flutnet;
using Flutnet.Interop.Embedding.Engine;
using Environment = System.Environment;
using MyFirstApp.ServiceLibrary;
namespace MyFirstApp
{
// Singleton class for Application wide objects.
public static class App
{
//.....
private static void ConfigureFlutnetRuntime()
{
try
{
// Init runtime
FlutnetRuntime.Init();
// Register My LoginService
FlutnetRuntime.RegisterPlatformService(new LoginService(), "login_service");
Initialized = true;
}
catch (Exception e)
{
// Handle init errors....
}
}
//.....
}
}
Xamarin iOS
ViewController.cs
using System;
using UIKit;
using Flutnet;
using Flutnet.Interop;
using MyFirstApp.ServiceLibrary;
using System.Threading.Tasks;
namespace MyFirstApp
{
public partial class ViewController : FlutterViewController
{
// ........
public override async void ViewDidAppear(bool animated)
{
base.ViewDidAppear(animated);
try
{
// Init runtime
FlutnetRuntime.Init();
// Register My LoginService
FlutnetRuntime.RegisterPlatformService(new LoginService(), "login_service");
// Start the bridge ....
}
catch (Exception e)
{
// Handle init errors
}
}
}
}
Flutter module project
Using the registration key you can obtain a reference of your instance.
import 'package:flutter/material.dart';
import 'package:my_first_app_bridge/my_first_app/service_library/login_service.dart';
// Somewhere in your Dart code....
// Reference to the xamarin login service
LoginService _loginService = LoginService("login_service");
try {
await _loginService.login(username: "admin", password: "admin"); // The best password ever ;-)
} catch (ex) {
// handle some other errors
}
What object you can register?
There is no check about the objects you register. If you register an invalid object, all his calls will fail. You should register only the objects marked with [PlatformService] attribute.
All the service must be declared or implemented in the Service Library Project (NET standard). If you need to implement specific platform code for a service class you should:
- define a public interface in the service module project (using [PlatformService] attribute)
- build your service module project and run flutnet pack on your compiled dll to generate the bridge_package (for flutter)
- implement the service interface in both Android or iOS projects, registering the specific instance in the FlutnetRuntime.
To understand why, just check the introduction about the Flutnet Architecture.
The image below show an example to how implement specific platform service using Flutnet: on the NET standard project you define your ServiceInterface using the specific PlatformService attribute. After that you can implement your service in Android and iOS project, registering the specific service on the FlutnetRuntime.
In this way Flutter module will reference an unique service, customized on the specific platform.
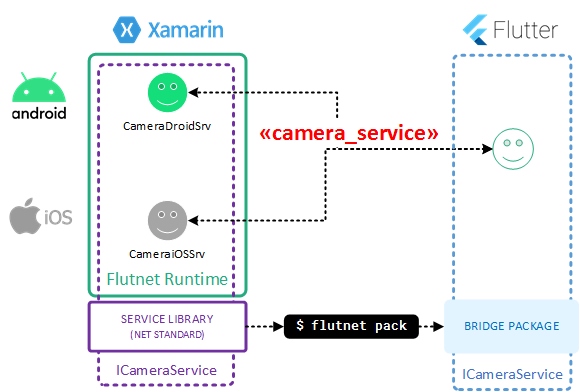